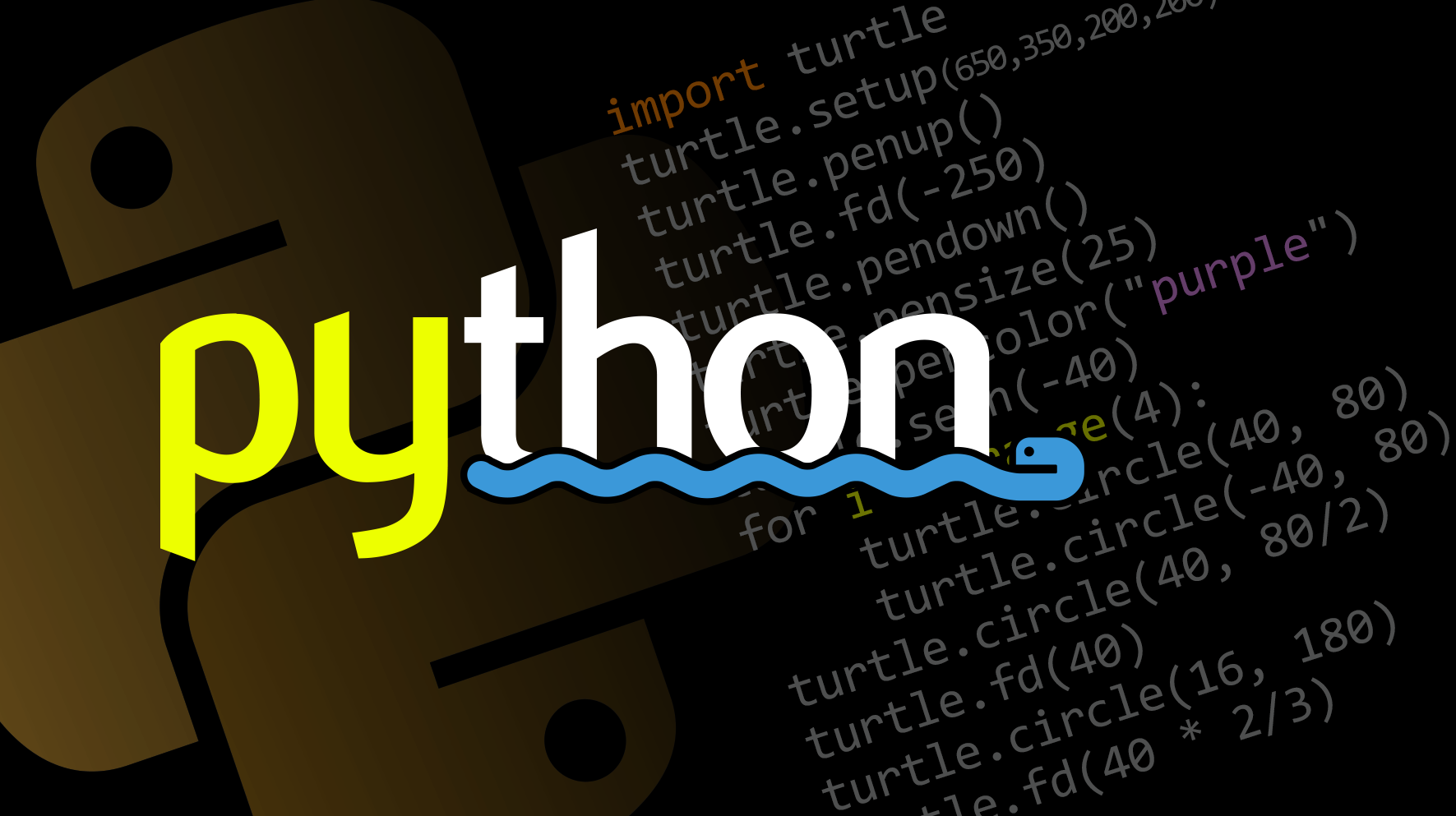
Python实验八——面向对象编程
创建类计算数学
1 | import math |
解释:
类定义:
class MyMath:
定义了一个名为MyMath
的类,表示几何计算的数学工具。**初始化方法
__init__
**:- 接收一个参数
radius
(半径)。 - 使用
self.radius = radius
将传入的radius
参数绑定到实例属性radius
上,供后续方法调用。 - 这样,每个
MyMath
对象都可以基于特定的半径进行计算。
- 接收一个参数
使用示例:
1 | circle = MyMath(radius=5) # 创建一个半径为 5 的实例 |
摄氏温度华氏温度转换
1 | class Temperature: |
不多解释了,和上面一样。
- 标题: Python实验八——面向对象编程
- 作者: W1ndys
- 创建于 : 2024-11-20 08:07:21
- 更新于 : 2025-02-13 08:04:58
- 链接: https://blog.w1ndys.top/posts/44cb2a.html
- 版权声明: 版权所有 © W1ndys,禁止转载。
评论